XGBoost: Quick Reference
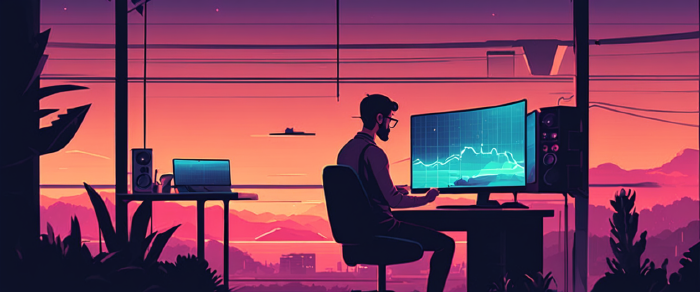
In the realm of machine learning algorithms, XGBoost stands out as a powerful tool for predictive modeling. Its efficiency, flexibility, and accuracy have made it a favorite among data scientists and machine learning practitioners. This note delves into what XGBoost is, how it works, and demonstrates its implementation using Python with illustrative examples.
XG What?
XGBoost, or eXtreme Gradient Boosting, is an open-source implementation of gradient boosting machines. It was developed by Tianqi Chen and is renowned for its scalability and speed. XGBoost is particularly effective in handling structured/tabular data and is widely used in various machine learning competitions and real-world applications.
How does XGBoost work?
XGBoost belongs to the family of ensemble learning methods, specifically boosting algorithms. It builds a strong predictive model by combining multiple weak learners sequentially. The key idea behind boosting is to focus on instances that are difficult to predict, thereby iteratively improving the model’s performance.
XGBoost employs a gradient boosting framework, wherein each weak learner is trained to minimize a loss function. It optimizes the overall objective by adding weak learners in a greedy manner, with each subsequent learner correcting the errors of its predecessors.
Gradient Boosting
Here’s how Gradient Boosting works:
-
Base Model Initialization: Gradient Boosting starts by initializing the model with a simple base model, often a decision tree with a fixed maximum depth (called a “weak learner”).
-
Initial Prediction: The base model makes initial predictions on the dataset.
-
Residual Calculation: The difference between the actual target values and the predictions made by the base model is calculated. This difference is known as the residual error.
-
Fitting a New Model to Residuals: A new model is then fit to predict these residuals. This model is chosen such that it corrects the errors made by the previous model.
-
Update Prediction: The predictions of the new model are combined with the predictions of the previous model to update the overall prediction. This is done by adding these predictions to the previous predictions.
-
Iteration: Steps 3 to 5 are repeated iteratively, with each new model attempting to correct the errors of the combined models from the previous iterations. The algorithm uses gradient descent to optimize the parameters of the base model in each iteration.
-
Final Prediction: The final prediction is made by combining the predictions of all the models in the ensemble.
Gradient Boosting differs from traditional ensemble methods like Random Forests in that it builds models sequentially, with each new model focusing on the errors made by the previous models. This sequential nature allows Gradient Boosting to improve upon the weaknesses of its predecessors, leading to highly accurate predictions.
Minimal Python Implementation
Let’s walk through a simple example to demonstrate how to use XGBoost in Python for a binary classification task.
Installation with conda:
conda install -c conda-forge py-xgboost
If the GPU autodetect fails, you can install using CPU/GPU specific options:
# CPU only
conda install -c conda-forge py-xgboost-cpu
# with a CUDA GPU
conda install -c conda-forge py-xgboost-gpu
Windows users will need Visual C++ Redistributable, that usually comes with Visual Studio installations.
Code
import xgboost as xgb
from sklearn.datasets import load_breast_cancer
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Loading dataset
data = load_breast_cancer()
X, y = data.data, data.target
# Splitting data into train and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Instantiating XGBoost classifier
clf = xgb.XGBClassifier()
# Training the model
clf.fit(X_train, y_train)
# Making predictions
y_pred = clf.predict(X_test)
# Evaluating model performance
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)